Learn multiple methods with Python code examples to easily list all files in a directory, including filtering and customization options.
This article explains how to use Python to list all files within a specific directory. We'll utilize the 'os' module, which allows interaction with the operating system's filesystem, and demonstrate how to filter the results to obtain a list of only files.
First, import the 'os' module. This module provides a way to interact with the operating system, including the filesystem.
To get a list containing all files and directories within a specific directory, use os.listdir(path)
. Replace 'path' with the actual path to the directory you're interested in. For example, to list the contents of the current directory, use os.listdir('.')
.
os.listdir()
returns a list of strings, where each string represents a file or directory name. It doesn't provide information about whether an item is a file or a directory.
To filter the list and get only files, you can use os.path.isfile(path)
. This function takes a path and returns True
if the path points to a file, and False
otherwise.
Combine os.listdir()
and os.path.isfile()
to list only files within a directory. Iterate through the items returned by os.listdir()
, and for each item, construct the full path by joining the directory path with the item name using os.path.join(directory, item)
. Then, use os.path.isfile()
to check if the constructed path points to a file. If it does, print the file name or store it in a separate list.
This Python code lists all files present in a specified directory. It uses the os module to interact with the file system, filters the directory contents to identify files, and then prints the names of those files.
import os
# Specify the directory path
directory_path = '/path/to/your/directory'
# Get the list of all files and directories
all_items = os.listdir(directory_path)
# Filter the list to get only files
files = []
for item in all_items:
# Construct the full path to the item
item_path = os.path.join(directory_path, item)
# Check if the item is a file
if os.path.isfile(item_path):
files.append(item)
# Print the list of files
print("Files in", directory_path + ":")
for file in files:
print(file)
Explanation:
os
module: This line imports the necessary module for interacting with the operating system./path/to/your/directory
with the actual path of the directory you want to list files from.os.listdir(directory_path)
returns a list of all files and directories within the specified directory.files
to store the file names.item
in the all_items
list.item
using os.path.join()
.os.path.isfile()
to check if the item_path
points to a file.item
(file name) to the files
list.files
list and print each file name.To run this code:
list_files.py
).python list_files.py
.This will print a list of all files present in the specified directory.
try-except
blocks to gracefully handle cases where the directory is not found or inaccessible./path/to/your/directory
). You can also use relative paths (e.g., 'my_folder/data'
) which are relative to the current working directory of your script.os.scandir()
: For Python versions 3.5 and above, os.scandir()
is generally more efficient than os.listdir()
as it returns directory entries with more information (like file type) without needing additional calls to os.path.isfile()
.os.walk()
.os.path.splitext()
function to extract the extension and compare it against your desired extensions.os
module provides functions like os.path.getsize()
and os.path.getmtime()
to retrieve file size and modification time, respectively. You can incorporate these if you need to filter or sort files based on these attributes.os
module provides cross-platform functionality, there might be subtle differences in how file paths are handled on different operating systems (Windows, macOS, Linux). Be mindful of these differences when writing code intended to run on multiple platforms.This text explains how to use Python's os
module to list all files within a specific directory:
Step | Description | Code Example |
---|---|---|
1. Import the os module |
Enables interaction with the operating system. | import os |
2. Get all files and directories | Use os.listdir() to get a list of all items within a directory. |
os.listdir('/path/to/directory') |
3. Filter for files | Use os.path.isfile() to check if an item is a file. |
os.path.isfile('/path/to/item') |
4. Combine and iterate | Iterate through the list from os.listdir() , construct the full path for each item using os.path.join() , and use os.path.isfile() to print or store only the files. |
```python |
import os | ||
directory = '/path/to/directory' | ||
for item in os.listdir(directory): | ||
full_path = os.path.join(directory, item) | ||
if os.path.isfile(full_path): |
print(item)
## Conclusion
In conclusion, this article provides a practical guide on listing files within a directory using Python's `os` module. By leveraging functions like `os.listdir()` and `os.path.isfile()`, you can efficiently retrieve and filter file names. The provided code snippet serves as a starting point, which can be further customized with error handling, path manipulation, and additional file attribute retrieval. Understanding these techniques empowers you to perform essential file system operations for various programming tasks.
## References
* 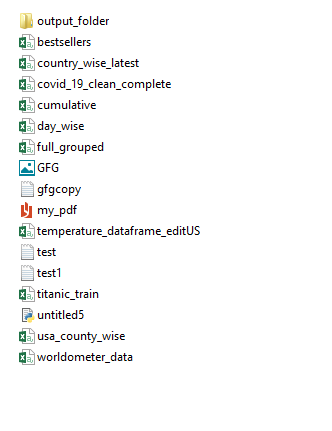 [Python - List Files in a Directory - GeeksforGeeks](https://www.geeksforgeeks.org/python-list-files-in-a-directory/) | A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
* 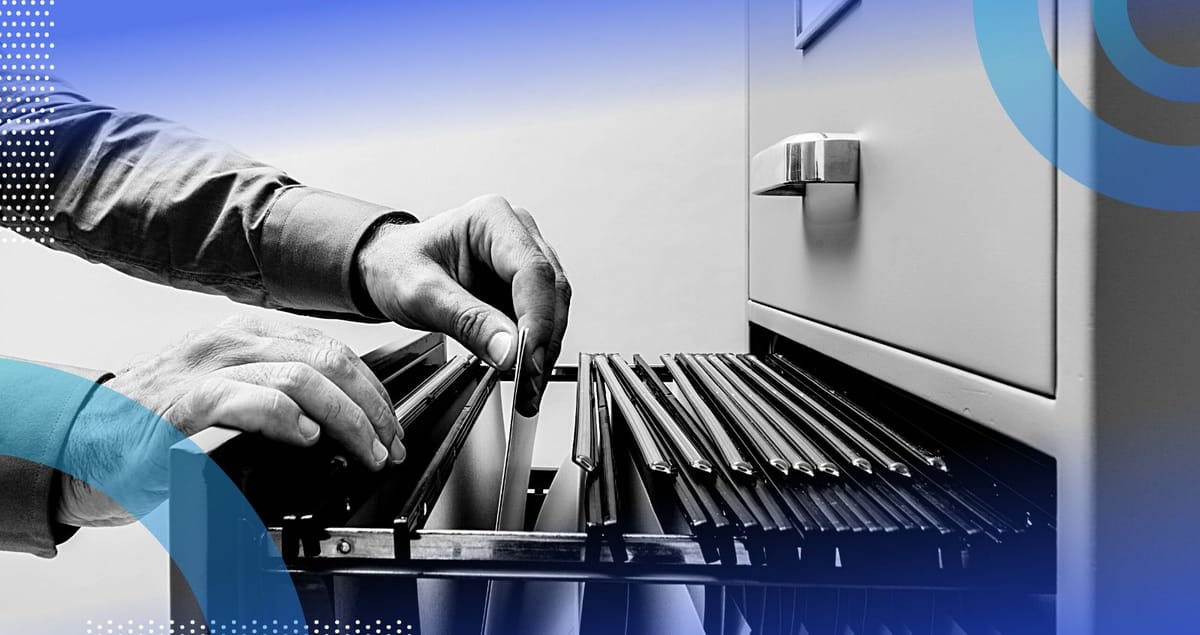 [Python: How to List Files in Directory | Built In](https://builtin.com/data-science/python-list-files-in-directory) | In this tutorial, you will learn 5 ways in Python to list all files in a specific directory. Methods include os.listdir(), os.walk(), the glob module and more.
* 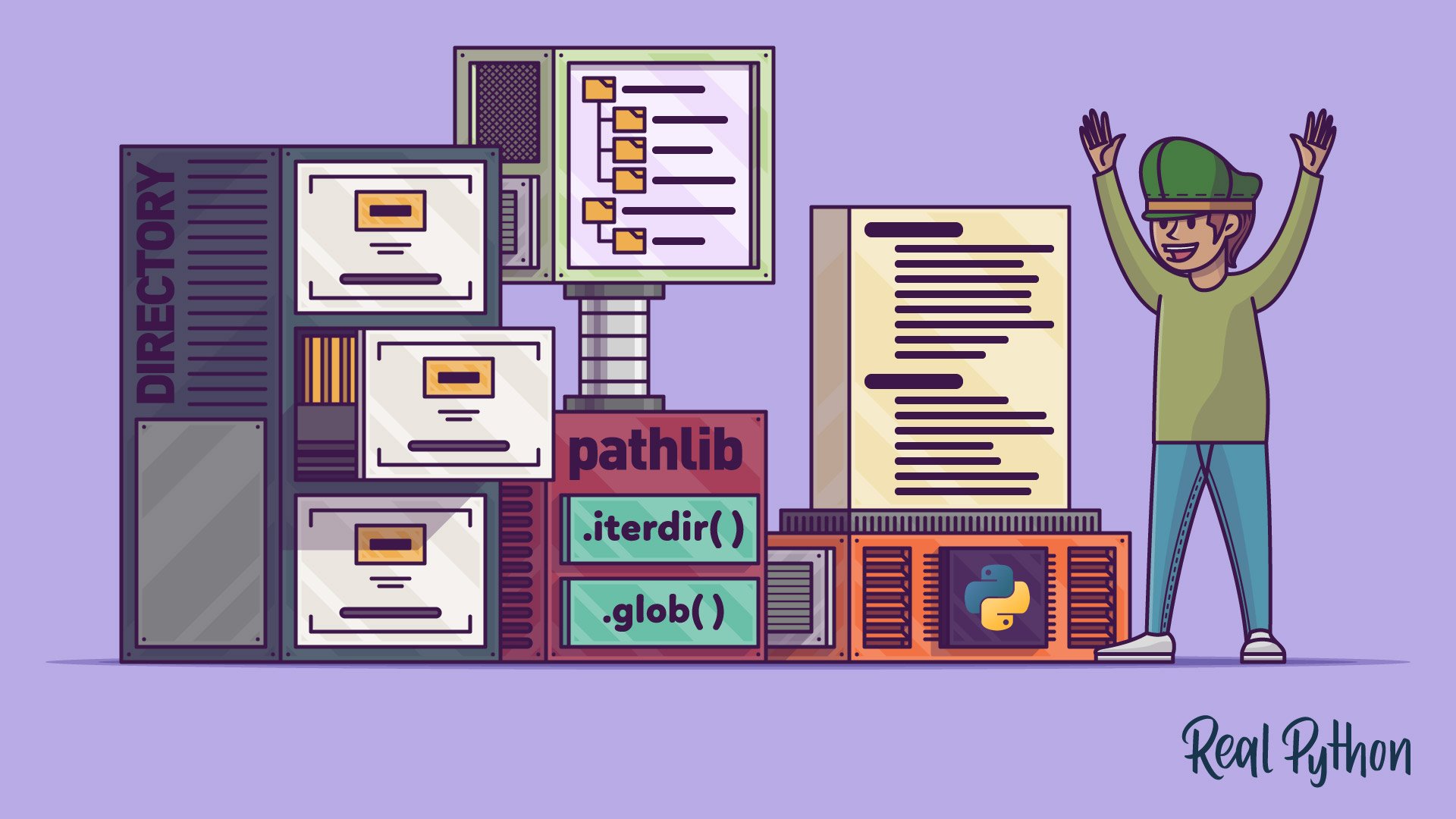 [How to Get a List of All Files in a Directory With Python – Real Python](https://realpython.com/get-all-files-in-directory-python/) | In this tutorial, you'll be examining a couple of methods to get a list of files and folders in a directory with Python. You'll also use both methods to recursively list directory contents. Finally, you'll examine a situation that pits one method against the other.
* 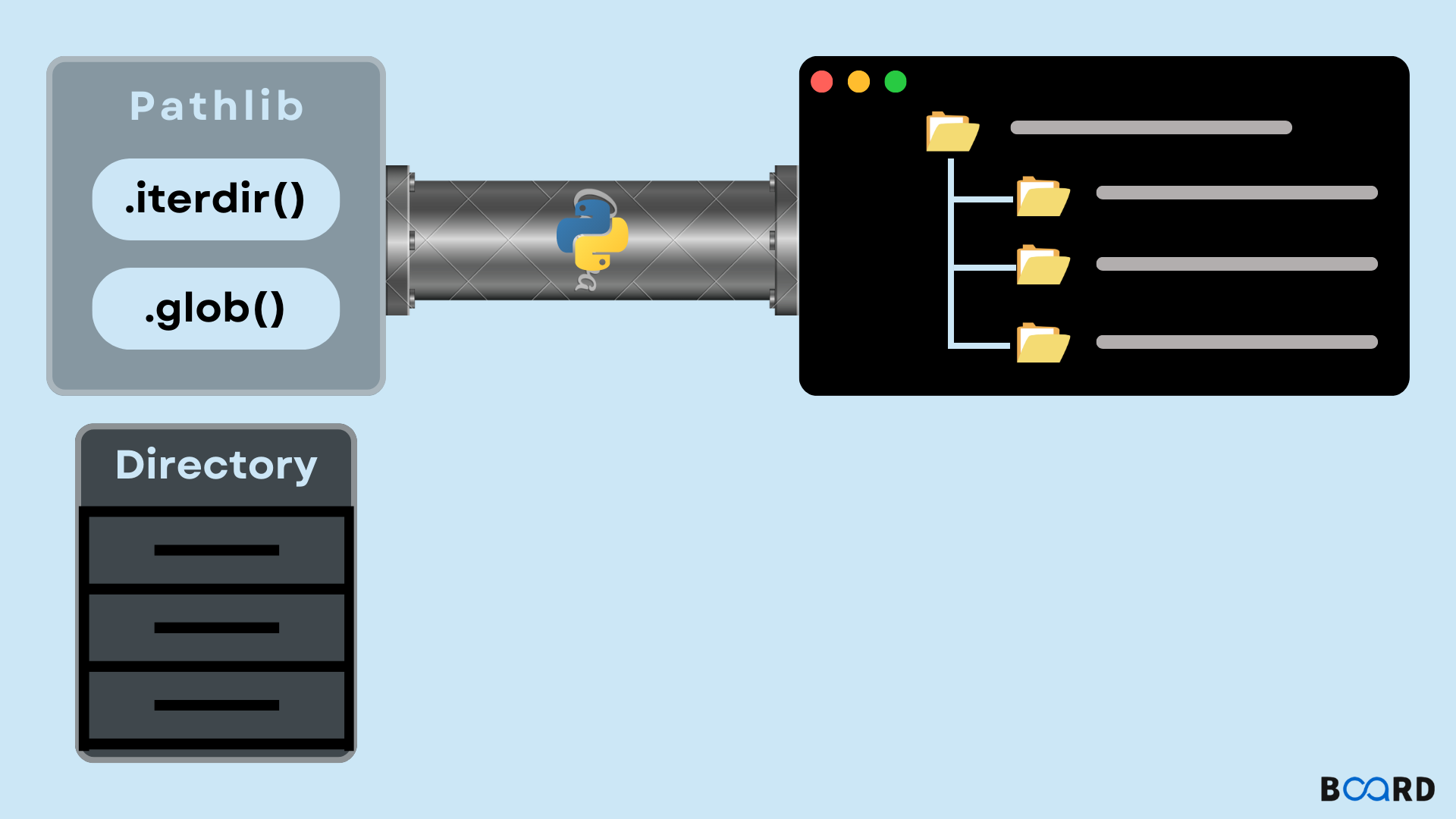 [List Files in a Directory in Python | Board Infinity](https://www.boardinfinity.com/blog/python-list-files-in-a-directory/) | This tutorial will look at how to list every file in a directory in Python. There are various approaches to documenting the files in a guide. The following four techniques will be used in this article.
* ![Python List Files in a Directory [5 Ways] – PYnative](/placeholder.png) [Python List Files in a Directory [5 Ways] – PYnative](https://pynative.com/python-list-files-in-a-directory/) | Get list of all files of a directory in Python using the the os module's listdir(), walk(), and scandir() functions
* 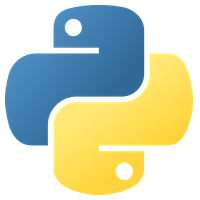 [pathlib — Object-oriented filesystem paths — Python 3.12.7 ...](https://docs.python.org/3/library/pathlib.html) | Source code: Lib/pathlib.py This module offers classes representing filesystem paths with semantics appropriate for different operating systems. Path classes are divided between pure paths, which p...
* 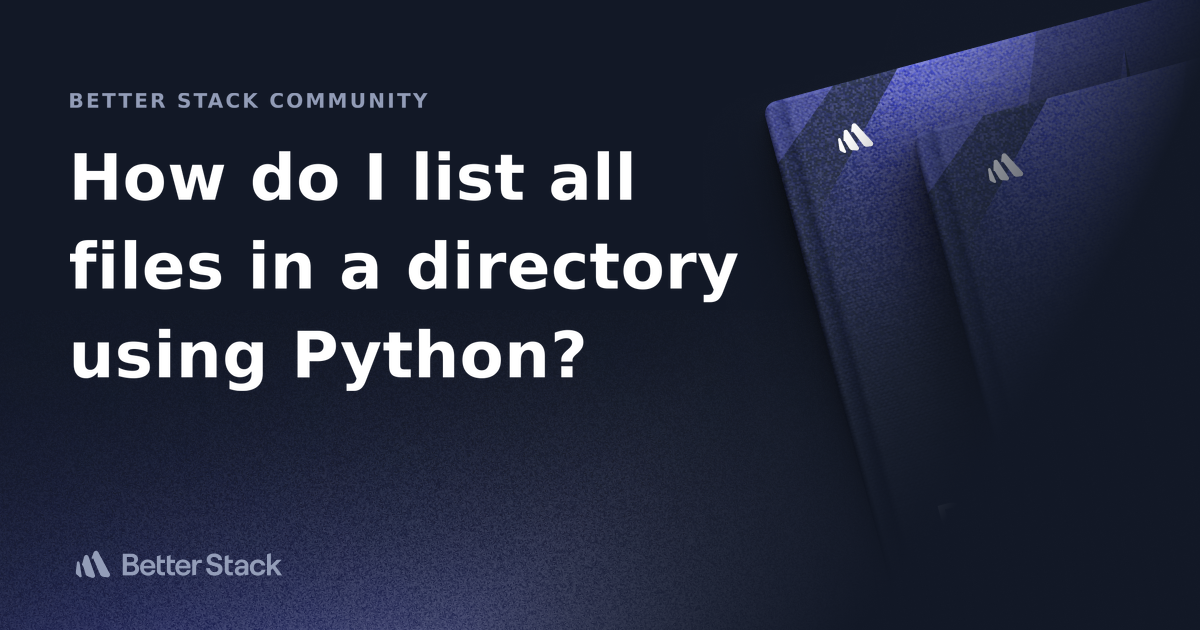 [How do I list all files in a directory using Python? | Better Stack ...](https://betterstack.com/community/questions/python-how-to-list-all-files-in-dictionary/) | Better Stack lets you see inside any stack, debug any issue, and resolve any incident.
* 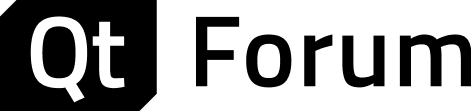 [How to read all files from a selected directory and use them one by ...](https://forum.qt.io/topic/64817/how-to-read-all-files-from-a-selected-directory-and-use-them-one-by-one) | Hi My main aim is to open a window to display some images of same size as a stack of images(sequence of images) and place a scroll bar such that when i move ...
* 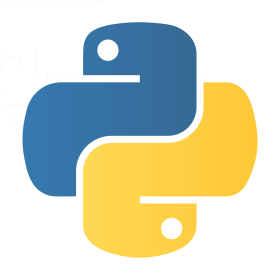 [Python - How to list all files in a directory? - Mkyong.com](https://mkyong.com/python/python-how-to-list-all-files-in-a-directory/) | - Python - How to list all files in a directory?