Learn how to accurately detect and track people in real-time using the powerful computer vision library OpenCV.
This article provides a step-by-step guide on how to perform people detection, tracking, and re-identification in video streams or images using Python and OpenCV. We will utilize the Histogram of Oriented Gradients (HOG) descriptor for detection, the CSRT algorithm for tracking, and deep learning models for re-identification.
import cv2
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
video_capture = cv2.VideoCapture(0) # For webcam
# or
image = cv2.imread('image.jpg')
(rects, weights) = hog.detectMultiScale(frame, winStride=(4, 4), padding=(8, 8), scale=1.05)
for (x, y, w, h) in rects:
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imshow('People Detection', frame)
cv2.waitKey(1)
tracker = cv2.TrackerCSRT_create()
tracker.init(frame, bounding_box)
(success, box) = tracker.update(frame)
person_reid.py
:model = cv2.dnn.readNetFromTorch('person_reid.pth')
features = model.forward(person_image)
This Python code uses OpenCV to perform real-time people detection and tracking in a video stream. It utilizes a pre-trained HOG descriptor for person detection and optionally employs a CSRT tracker to follow detected individuals across frames. The code captures video from the default camera, detects people within each frame, and draws bounding boxes around them. If a tracker is initialized, it updates the position of the tracked person. The resulting video output displays the original frames with bounding boxes highlighting detected people. The program terminates when the 'q' key is pressed.
# Import necessary libraries
import cv2
# Load pre-trained HOG descriptor
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# Open video stream
video_capture = cv2.VideoCapture(0)
# Initialize tracker (optional)
tracker = None
while True:
# Read frame from video stream
ret, frame = video_capture.read()
# Detect people in the frame
(rects, weights) = hog.detectMultiScale(frame, winStride=(4, 4),
padding=(8, 8), scale=1.05)
# Draw bounding boxes around detected people
for (x, y, w, h) in rects:
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
# Initialize tracker if not already initialized
if tracker is None:
tracker = cv2.TrackerCSRT_create()
tracker.init(frame, (x, y, w, h))
# Update tracker if initialized
if tracker is not None:
(success, box) = tracker.update(frame)
if success:
(x, y, w, h) = [int(v) for v in box]
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 0, 255), 2)
# Display the output
cv2.imshow('People Detection', frame)
# Break the loop if 'q' key is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the video capture object
video_capture.release()
cv2.destroyAllWindows()
Explanation:
detectMultiScale
method of the HOG descriptor to detect people in the current frame.Note:
person_reid.py
as mentioned in the article.detectMultiScale
method to fine-tune the detection accuracy.0
in cv2.VideoCapture(0)
with the path to your video file.## Additional Notes
**General:**
* **Performance:** HOG + SVM is relatively fast for person detection, but can be computationally expensive for real-time applications, especially with high-resolution video. Consider downsampling frames or using a more efficient detector if needed.
* **Accuracy:** Detection accuracy depends heavily on the quality of the video stream, lighting conditions, and the diversity of the training data used for the HOG descriptor.
* **False Positives:** The algorithm might generate false positives, detecting objects that are not people. Fine-tuning parameters and potentially training a custom HOG descriptor on relevant data can help mitigate this.
**Detection (HOG):**
* **`detectMultiScale` parameters:** Experiment with different values for `winStride`, `padding`, and `scale` to optimize for your specific scenario (e.g., smaller stride for detecting smaller people).
* **Custom HOG Descriptor:** For improved accuracy on specific datasets or scenarios, consider training your own HOG descriptor using positive and negative samples.
**Tracking (CSRT):**
* **Tracker Initialization:** The tracker needs to be re-initialized whenever the person is lost or a new person enters the scene. This can be done by detecting people in each frame and associating them with existing tracks.
* **Occlusion Handling:** The tracker might lose track of a person if they are occluded for a long time. Implement strategies like track prediction or re-identification to handle occlusions.
* **Other Tracking Algorithms:** Explore other tracking algorithms like KCF, MIL, or deep learning-based trackers for potentially better performance depending on your needs.
**Re-Identification:**
* **Deep Learning Models:** Pre-trained models for person re-identification are available in libraries like OpenCV. You can also fine-tune these models on your own dataset for improved accuracy.
* **Feature Extraction:** The `person_reid.py` example in OpenCV demonstrates how to extract features from person images using a deep learning model. These features can then be used for similarity comparison.
* **Similarity Metrics:** Use appropriate distance metrics like cosine similarity or Euclidean distance to compare feature vectors and determine if two detections belong to the same person.
**Additional Features:**
* **Counting People:** Implement a counter to track the number of people entering or leaving a specific area.
* **Activity Recognition:** Analyze the movement patterns of tracked people to recognize actions like walking, running, or falling.
* **Privacy Considerations:** Be mindful of privacy concerns when implementing people tracking systems. Consider anonymizing or discarding personal data appropriately.
## Summary
This code snippet demonstrates how to detect and track people in images or videos using OpenCV in Python.
**Key Steps:**
1. **Load pre-trained HOG descriptor:** Utilize OpenCV's pre-trained Histogram of Oriented Gradients (HOG) descriptor and Support Vector Machine (SVM) classifier for efficient pedestrian detection.
2. **Capture input:** Access live video streams from webcams or load static images from files.
3. **Detect people:** Apply the HOG descriptor to identify potential human figures within each frame or image, generating bounding boxes around them.
4. **Visualize detections:** Draw rectangles around detected individuals in real-time, providing a visual representation of the algorithm's output.
5. **Track individuals:** Implement object tracking algorithms like CSRT (Channel and Spatial Reliability Tracking) to follow specific people across consecutive frames, maintaining their identities even when they move.
6. **Re-identify individuals:** For advanced scenarios, leverage deep learning models and libraries like OpenCV's `person_reid.py` to extract unique features from detected individuals. This enables re-identification of the same person across different cameras or time periods by comparing feature similarity scores.
**Libraries Used:**
- `cv2` (OpenCV)
**Key Functions:**
- `cv2.HOGDescriptor()`
- `cv2.HOGDescriptor_getDefaultPeopleDetector()`
- `hog.detectMultiScale()`
- `cv2.TrackerCSRT_create()`
- `cv2.dnn.readNetFromTorch()`
This approach provides a foundation for building applications requiring pedestrian detection, tracking, and re-identification, such as security systems, crowd analysis, and retail analytics.
## Conclusion
This article explored techniques for people detection, tracking, and re-identification in video streams or images using Python and OpenCV. By leveraging the HOG descriptor for detection, the CSRT algorithm for tracking, and deep learning models for re-identification, developers can build robust applications for various purposes. The code example provided offers a starting point for implementing these techniques, while the discussion of parameters, alternative algorithms, and potential challenges empowers readers to further refine and adapt the code to their specific needs. As computer vision technology continues to advance, we can expect even more sophisticated and efficient methods for people detection and tracking to emerge, opening up new possibilities in fields like security, retail, and human-computer interaction.
## References
* 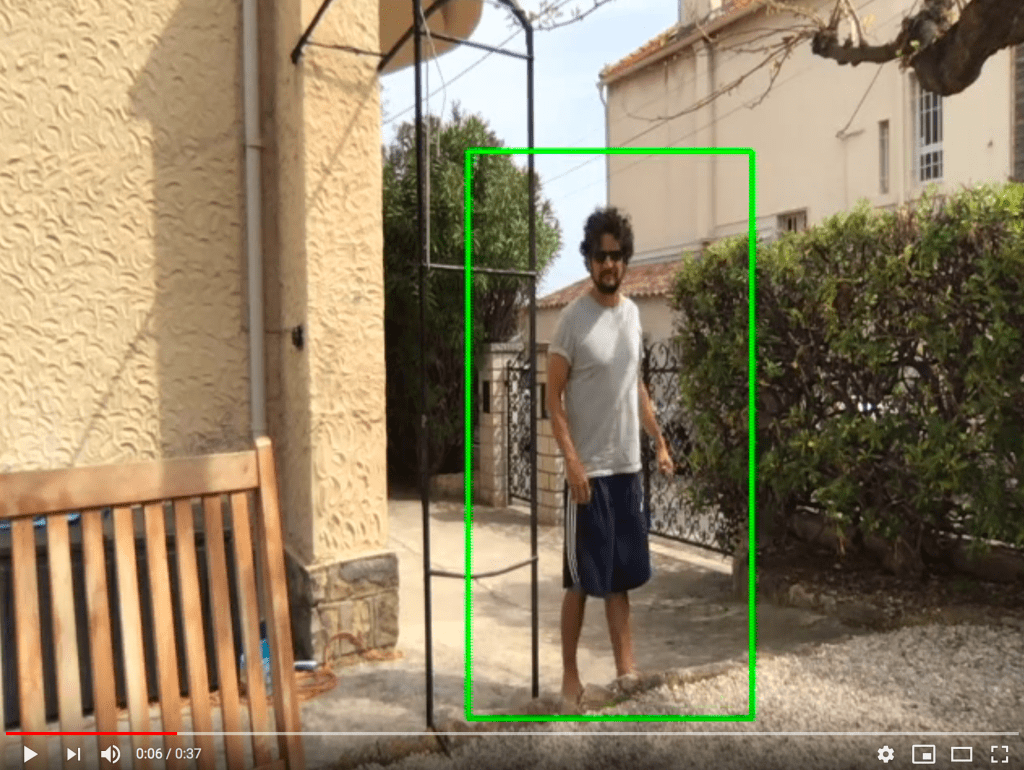 [Real-time Human Detection with OpenCV](https://thedatafrog.com/en/articles/human-detection-video/) | Let's use the HOG algorithm implemented in OpenCV to detect people in real time in a video stream!
* 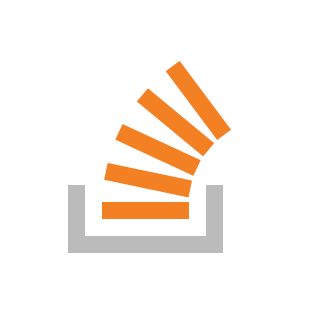 [image processing - Using OpenCV to find people who wear a ...](https://stackoverflow.com/questions/15204391/using-opencv-to-find-people-who-wear-a-certain-hat) | Mar 4, 2013 ... The first link you posted describes a simple color-based detection. You can try that, but it will fail if there are other pixel clusters of similar color inĀ ...
* 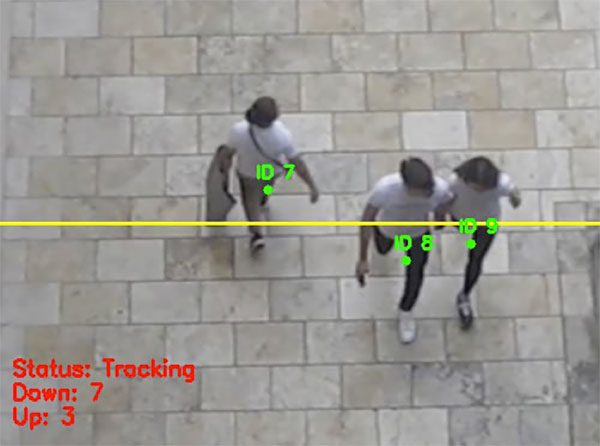 [OpenCV People Counter - PyImageSearch](https://pyimagesearch.com/2018/08/13/opencv-people-counter/) | This tutorial will teach you how to build a people counter using OpenCV, Python, and object tracking algorithms. Using the OpenCV library we'll count the number of people moving "in" and "out" of a store.
*  [How do I track the same person across multiple cameras? : r ...](https://www.reddit.com/r/computervision/comments/186lq7m/how_do_i_track_the_same_person_across_multiple/) | Posted by u/Puzzleheaded_Win9283 - 0 votes and 14 comments
*  [Person ReID in real time - Python - OpenCV](https://forum.opencv.org/t/person-reid-in-real-time/5795) | There are many ReID codes for OpenCV Python on the internet, but this is apparently the official one: opencv/person_reid.py at master Ā· opencv/opencv Ā· GitHub My question is: how do I make it real time? That is, I indicate a query and in real time I traced the person by indicating an ID, and that recognizes who the person at all times (because it currently recognizes the person of the query, but only generates a photo with all the appearances that it has in The galery). Can you help me?
* 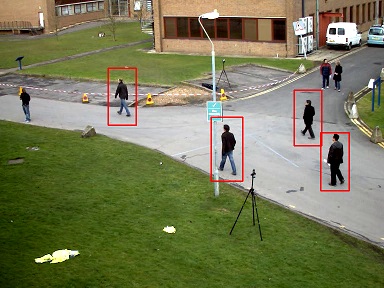 [How to record the time of stay by detected people in a video? edit](https://answers.opencv.org/question/80580/how-to-record-the-time-of-stay-by-detected-people-in-a-video/) | Dec 24, 2015 ... Maybe i will try to improve it or some ideas will raise. i tested it with 768x576.avi can be found OpenCV's \samples\data. ( tracking is notĀ ...
*  [Seeking Guidance for Developing a Gender-Sensitive People ...](https://forum.opencv.org/t/seeking-guidance-for-developing-a-gender-sensitive-people-counter-using-body-detection/17057) | Hello OpenCV Community, I am currently working on a project to develop a people counter system that not only tracks entrances and exits but also incorporates gender recognition. The primary challenge Iām facing is the need to rely on body detection for this task, as the camera setup does not consistently capture faces with sufficient quality for reliable facial recognition. The pedestriansā full bodies are visible, which I believe could be leveraged for gender determination. Given these constr...
*  [key-point based detection vs HoG/Haar training - OpenCV Q&A Forum](https://answers.opencv.org/question/29964/key-point-based-detection-vs-hoghaar-training/) | Mar 14, 2014 ... Also, people use different detectors specific for the object. For ... tracking algorithm here for example: Matrioska: Tracking By detection usingĀ ...
*  [Detecting small attachments on the body - Python - OpenCV](https://forum.opencv.org/t/detecting-small-attachments-on-the-body/7339) | Hi, Iām trying to detect some small attachments to the human body where the person wears minimal cloth. So, these attachments are directly on the skin. Iāve tried color filtering to reveal these small attachments but it really did not work since color of the attachments are not too far away from what is present in the image Also, Hough circles extracted too many circles. Another thing, I tried is feature extraction by SIFT. It extracts too many features that are hard to choose from. How can...