Learn how to troubleshoot and resolve the frustrating "Error: spawn ENOENT" in your Node.js applications.
The "Error: spawn ENOENT" in Node.js means the system can't find the file you're trying to run with child_process.spawn()
. This guide helps you troubleshoot this error. We'll break down the error message, check your code for mistakes in the command name, paths, or arguments, and then look at your system's environment to ensure the executable is accessible. Finally, you'll learn how to handle these errors gracefully in your code.
The "Error: spawn ENOENT" in Node.js signifies that the system cannot find the executable file you're trying to run using child_process.spawn()
. Here's a breakdown of how to debug this error:
Step 1: Understand the Error Message
child_process.spawn()
function, which is used to execute external commands.Step 2: Verify Your Code
Correct Command Name: Double-check that the command you're trying to execute is spelled correctly and exists within your system's PATH.
const { spawn } = require('child_process');
// Incorrect command name (assuming 'nood' is a typo)
const command = 'nood';
// Correct command name
// const command = 'node';
const process = spawn(command, ['-v']);
process.on('error', (err) => {
console.error(`Error: ${err.message}`);
});
Absolute vs. Relative Paths: If you're not relying on the system PATH, provide the full absolute path to the executable. Relative paths can be problematic if your Node.js process's working directory is different from what you expect.
const { spawn } = require('child_process');
// Relative path (potentially problematic)
// const command = './my-script.sh';
// Absolute path (more robust)
const command = '/usr/bin/my-script.sh';
const process = spawn(command);
Command Arguments: Ensure that any arguments passed to the command are correct and in the expected format.
const { spawn } = require('child_process');
const command = 'ls';
// Incorrect arguments (missing space)
// const args = ['-lafolder'];
// Correct arguments
const args = ['-la', 'folder'];
const process = spawn(command, args);
Step 3: Investigate System Environment
PATH Environment Variable: Verify that the directory containing your executable is included in your system's PATH environment variable. You can check this in your terminal:
echo $PATH
echo %PATH%
File Existence: Use the appropriate command to confirm the executable file actually exists at the path you specified:
ls -l /path/to/your/executable
dir /path/to\your\executable
Step 4: Handle Errors Gracefully
Error Event: Always listen for the 'error' event on the spawned child process to catch and handle ENOENT errors gracefully.
const { spawn } = require('child_process');
const process = spawn('your-command', ['your-args']);
process.on('error', (err) => {
if (err.code === 'ENOENT') {
console.error(`Executable not found: ${err.message}`);
// Implement error handling, e.g., provide a user-friendly message
} else {
console.error(`Error spawning process: ${err.message}`);
}
});
Additional Tips:
console.log
statements to inspect the values of variables like the command path and arguments before calling spawn()
.child_process.exec()
instead of spawn()
.By following these steps, you should be able to effectively debug and resolve "Error: spawn ENOENT" issues in your Node.js applications.
The JavaScript code demonstrates how to spawn child processes using the spawn
function from the child_process
module. It provides examples of common errors encountered when spawning processes, such as incorrect command names, relative path issues, and incorrect command arguments. The code also showcases how to handle the "ENOENT" error (executable not found) gracefully by listening for the 'error' event and implementing appropriate error handling.
const { spawn } = require('child_process');
// Example 1: Incorrect command name
try {
const process = spawn('nood', ['-v']); // Assuming 'nood' is a typo
process.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
} catch (err) {
console.error(`Error: ${err.message}`); // This will likely throw an ENOENT error
}
// Example 2: Relative path issue (assuming script is in the same directory)
try {
const process = spawn('./my-script.sh');
process.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
} catch (err) {
console.error(`Error: ${err.message}`); // Might throw ENOENT if working directory is different
}
// Example 3: Incorrect command arguments
try {
const process = spawn('ls', ['-lafolder']); // Missing space between '-la' and 'folder'
process.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
} catch (err) {
console.error(`Error: ${err.message}`); // Might throw an error depending on the command
}
// Example 4: Handling ENOENT gracefully
const command = 'my-command';
const args = ['arg1', 'arg2'];
const process = spawn(command, args);
process.on('error', (err) => {
if (err.code === 'ENOENT') {
console.error(`Executable not found: ${command}`);
// Implement error handling, e.g., provide a user-friendly message
} else {
console.error(`Error spawning process: ${err.message}`);
}
});
process.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
Explanation:
nood
) to demonstrate the ENOENT error.Remember:
'my-script.sh'
, 'my-command'
, 'arg1'
, etc., with your actual command, script name, and arguments.console.log
statements to inspect the values of variables like command
and args
before calling spawn()
to help with debugging.Here are some extra points to keep in mind when troubleshooting this error:
Context is Key:
process.cwd()
if you're working with relative paths.spawn()
if they depend on shell-specific features (e.g., aliases, environment variables set only in your shell profile).Going Deeper:
execFile()
for Direct Execution: If you're certain about the executable's location and don't need shell features, child_process.execFile()
might be a more direct alternative to spawn()
./
vs. \
) and potential differences in command names or locations when writing code that needs to run on both Windows and Unix-like systems.Best Practices:
Example: Enhanced Error Handling
const { spawn } = require('child_process');
const command = 'my-command';
const args = ['arg1', 'arg2'];
const process = spawn(command, args);
process.on('error', (err) => {
if (err.code === 'ENOENT') {
console.error(`Could not find the command '${command}'.
Please check the command name and your system's PATH.
Current working directory: ${process.cwd()}`);
} else {
console.error(`Error running command: ${err.message}`);
}
});
This enhanced example provides more informative error messages, including the command name and the current working directory, making it easier to pinpoint the source of the problem.
This table summarizes how to troubleshoot the "Error: spawn ENOENT" error, which occurs when Node.js cannot find the executable file you're trying to run with child_process.spawn()
:
Step | Description | Actions | Code Example |
---|---|---|---|
1. Understand the Error | Identify the meaning of "ENOENT" and "spawn" in the error message. | - ENOENT: "Error NO ENTry" - file/directory not found. - spawn: Error originates from child_process.spawn() . |
N/A |
2. Verify Your Code | Check for common code-related issues. | - Command Name: Verify spelling and existence in PATH. - Paths: Use absolute paths for robustness. - Arguments: Ensure correct format and spacing. |
```javascript |
// Correct command name and arguments: | |||
const command = 'node'; | |||
const args = ['-v']; | |||
const process = spawn(command, args); |
// Absolute path: const command = '/usr/bin/my-script.sh'; const process = spawn(command);
| **3. Investigate System Environment** | Examine system-level settings that might cause the error. | - **PATH Variable:** Confirm the executable's directory is in the PATH. <br> - **File Existence:** Verify the executable exists at the specified path. | - Check PATH: <br> - Linux/macOS: `echo $PATH` <br> - Windows: `echo %PATH%` <br> - Check file existence: <br> - Linux/macOS: `ls -l /path/to/your/executable` <br> - Windows: `dir /path/to\your\executable` |
| **4. Handle Errors Gracefully** | Implement error handling to catch and manage ENOENT errors. | - Listen for the 'error' event on the spawned child process. <br> - Implement specific error handling for ENOENT. | ```javascript
process.on('error', (err) => {
if (err.code === 'ENOENT') {
console.error(`Executable not found: ${err.message}`);
// Handle error (e.g., user-friendly message)
} else {
console.error(`Error spawning process: ${err.message}`);
}
});
``` |
| **Additional Tips** | Consider these extra debugging and troubleshooting techniques. | - Use debugging tools or `console.log` for inspection. <br> - Utilize `child_process.exec()` for shell environment execution. <br> - Verify Node.js process permissions for file execution. | N/A |
## Conclusion
Mastering the "Error: spawn ENOENT" in Node.js boils down to understanding that your system can't locate the executable you're trying to run. By meticulously checking your code for typos, ensuring accurate paths, and confirming your system environment, you can resolve this error effectively. Remember to implement robust error handling to gracefully manage these situations and provide helpful feedback to users. With these strategies, you can confidently tackle "Error: spawn ENOENT" and keep your Node.js applications running smoothly.
## References
*  [Build failing with "spawn bundle ENOENT" error · vercel · Discussion ...](https://github.com/orgs/vercel/discussions/5424) | Question Hello ! Since ~30 minutes, it seems my deployments are failing, just after a git clone has been successfully done: Example: Running build in Washington, D.C., USA (East) – iad1 13:38:06.02...
* 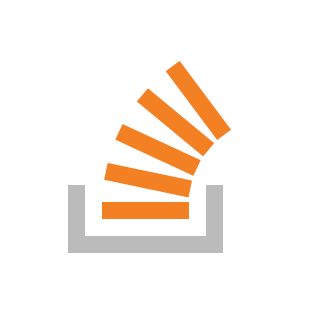 [node.js - How to catch an ENOENT with nodejs child_process ...](https://stackoverflow.com/questions/34208614/how-to-catch-an-enoent-with-nodejs-child-process-spawn) | Dec 10, 2015 ... Similar but different: How do I debug "Error: spawn ENOENT" on node.js? I know exactly why I am getting ENOENT, and I know what to change to ...
*  [Error: spawn ENOENT on Ubuntu 14.04 · Issue #708 · Unitech/pm2 ...](https://github.com/Unitech/pm2/issues/708) | Hi, I'm getting the following error on Ubuntu 14.04 for almost anything I try to do with pm2: Starting PM2 daemon... events.js:72 throw er; // Unhandled 'error' event ^ Error: spawn ENOENT at errno...
* 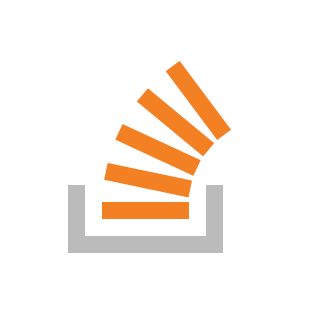 [node.js - Error: spawn ENOENT on Windows - Stack Overflow](https://stackoverflow.com/questions/37459717/error-spawn-enoent-on-windows) | May 26, 2016 ... How do I debug "Error: spawn ENOENT" on node.js? 0 · AWS Lambda nodejs function throwing exception to generate html to pdf using html-pdf · 1.
*  [`Error: spawn ENOENT` when executing a program installed ...](https://github.com/nodejs/node-v0.x-archive/issues/5841) | When I install an npm module globally, I'm able to execute it in a child process with the exec method, but not with spawn. So, this will print the version of Express (although sometimes it won't pr...
* 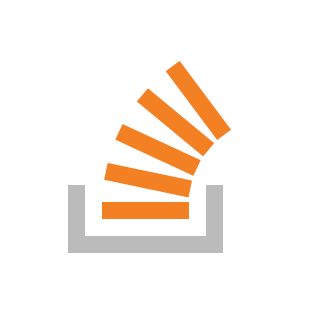 [javascript - Node js Error: spawn ENOENT - Stack Overflow](https://stackoverflow.com/questions/18846918/node-js-error-spawn-enoent) | Sep 17, 2013 ... Node js Error: spawn ENOENT ... I have specified the path for nodejs. But i dont know why it fails. Any idea about this issue? javascript · node.
*  [karma won't launch any browser ENOENT · Issue #479 · karma ...](https://github.com/karma-runner/karma/issues/479) | I'm runnning Windows 7 x64 node.js 0.10.3 karma 0.8.4 I've set all my *_BIN ENV variables, `echo %CHROME_BIN% "C:\Program Files (x86)\Google\Chrome\Application\chrome.exe"`` I've checked the paths ...
* 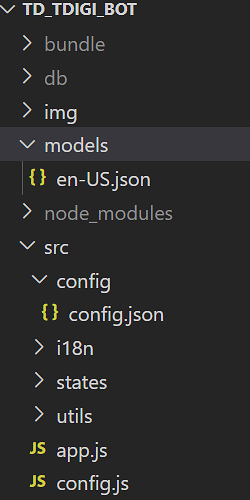 [Error Message "ENOENT" - Questions - Jovo Community](https://community.jovo.tech/t/error-message-enoent/1662) | ENOENT No such file or directory. The specified file or directory does not exist or cannot be found. This message can occur whenever a specified file does not exist or a component of a path does not specify an existing directory. Would anybody have an idea where I can find a reference to the missing directory or the missing file? I’m brand new to Jovo and man, am I impressed with the product. EDIT: I was reading earlier references related to this issue of mine and I noticed that I do not hav...
*  [spawn error ENOENT ? · Issue #66 · workshopper/learn-sass · GitHub](https://github.com/workshopper/learn-sass/issues/66) | D:\Nodejs\sass>learn-sass verify stylesheet.scss Result --> events.js:137 throw er; // Unhandled 'error' event ^ Error: spawn C:\Users\pauli\AppData\Roaming\npm\node_modules\learn-sass\node_modules...